The following example demonstrates the use of RMSis API's which is used to create a report in MS-Word after dynamically fetching the data from RMsis using macros.
Pre-Requisites:
- RMsis version v1.8.9.2 or higher.
- MS-Word with developer module to write macro.
Following steps were taken:
- Populate data in RMsis.
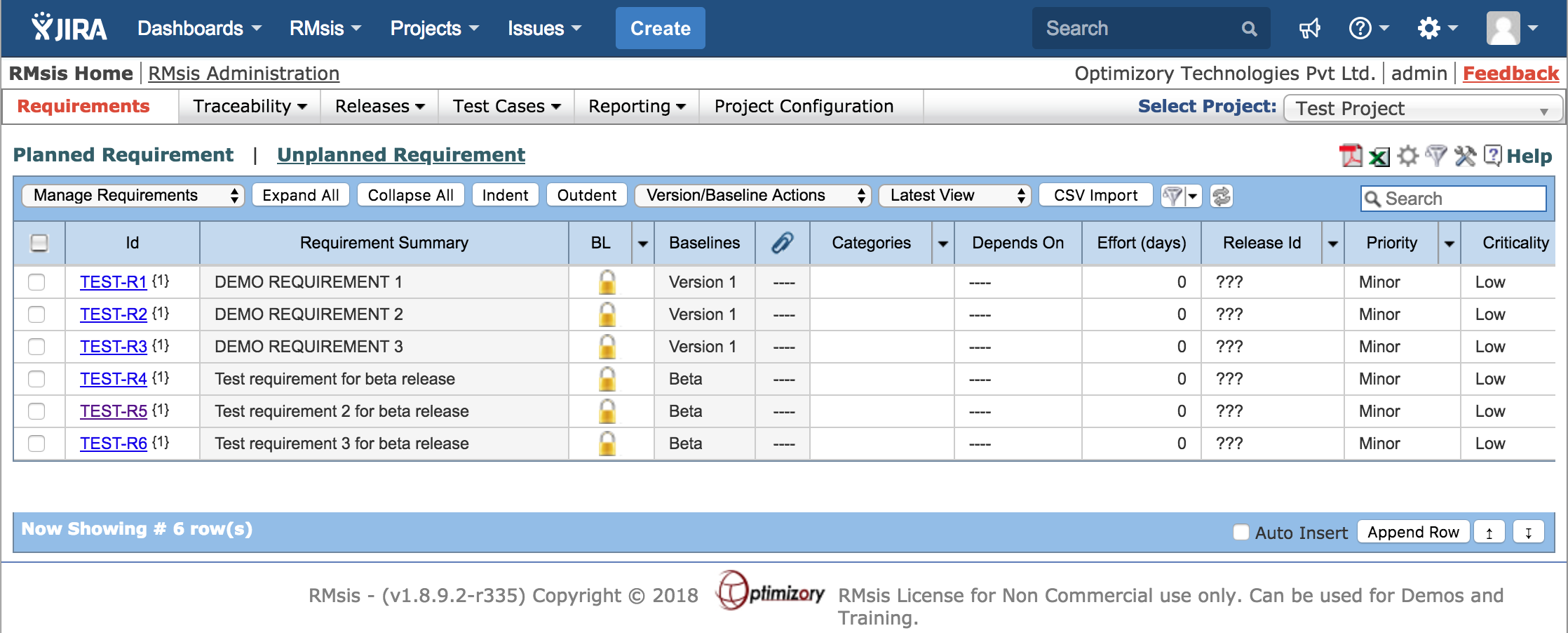
The above RMsis screen displays list of requirements present in RMsis which have been baselined.
Note the two baselines present under the Baselines column, namely Version 1 and Beta.
- Create macro in MS-Word to fetch the data from RMsis and populate it into the word document. See attached file macroToFetchBaselineDetails.docm
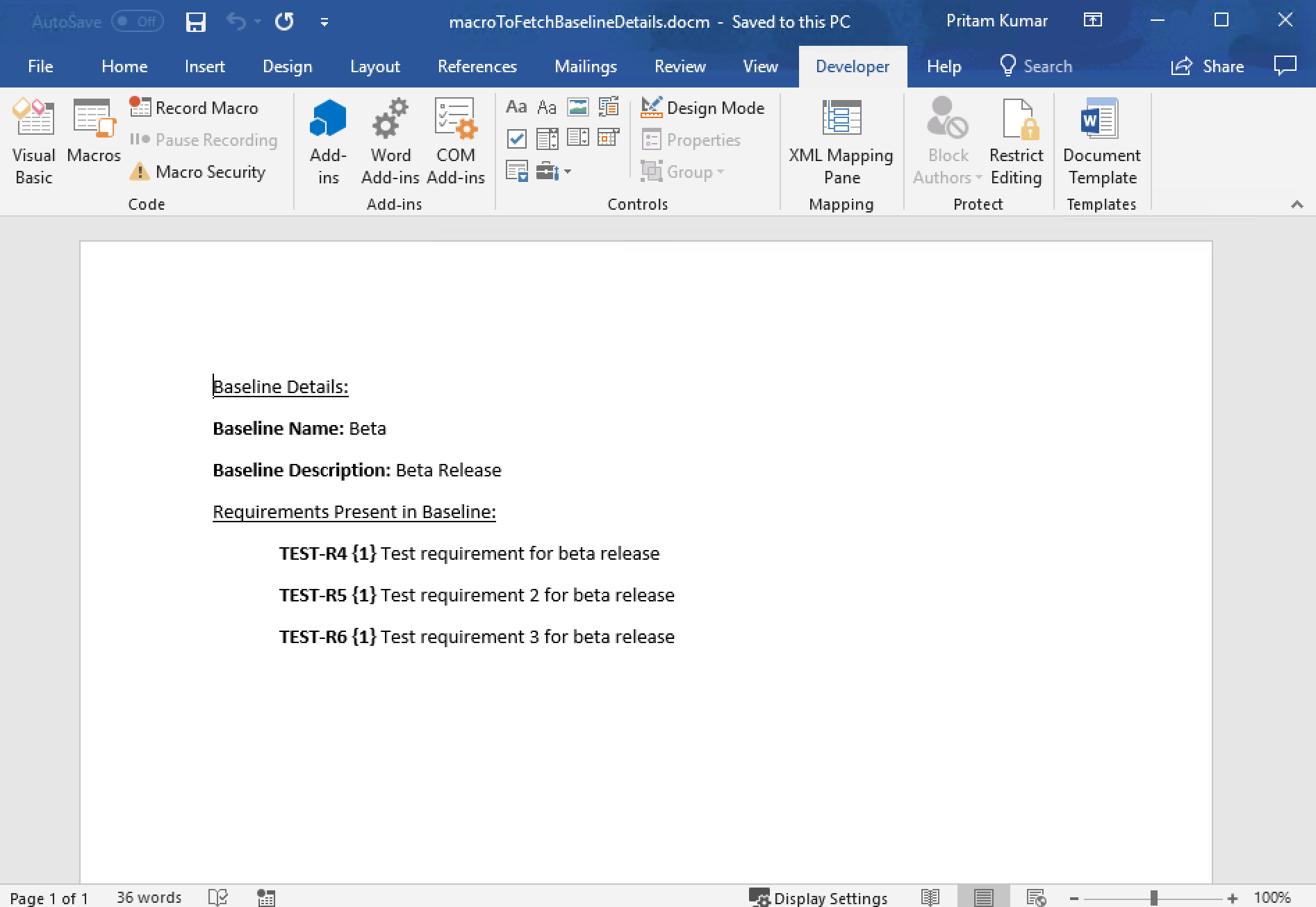
The word document created in MS word which lists the details of the baseline named "Beta".
The content is populated dynamically using word macro after fetching it from RMsis.
The code of the word macro is mentioned below, it contains following steps:
- Login into JIRA using a valid username and password.
- Fetch data from RMsis using API's.
- Insert the formatted data into word document.
Public restRequest
Sub loginAndFetchBaselineDetails()
Dim url, auth, responseStr
Set restRequest = CreateObject("Msxml2.ServerXMLHTTP.6.0")
url = "http://10.0.1.10:8080/rest/api/2/application-properties"
'the following base64 authentication string was created using a base64 conversion site(base64encode.org) as per the "username:password" format
'auth = "(base64 encoded <username:password>)" here "YWRtaW46YWRtaW4=" corresponds to "admin:admin"
auth = "(YWRtaW46YWRtaW4=)"
restRequest.Open "GET", url, False
restRequest.setRequestHeader "Authorization", "Basic " & auth
restRequest.send
'Check Jira Login
If restRequest.Status = "401" Then
MsgBox "Not authorized"
Else
'MsgBox "Authentication Successful"
responseStr = restRequest.responseText
End If
'RMsis graph api to fetch data of baseline beta whose id is 5 in RMsis
rmsisApiString = "{getBaselineById(id:5){description name}}"
responseText = GetRequestfromRMsis(rmsisApiString)
'Selection.TypeText Text:=GetRequestfromRMsis(rmsisApiString)
Dim baselineName, baselineDescription As String
Set JSON = ParseJson(responseText)
baselineName = JSON("data")("getBaselineById")("name")
baselineDescription = JSON("data")("getBaselineById")("description")
'Insert formatted baseline details in word document
Selection.Font.underline = wdUnderlineSingle
Selection.TypeText Text:="Baseline Details:"
Selection.Font.underline = wdUnderlineNone
Selection.TypeParagraph
Selection.Font.bold = wdToggle
Selection.TypeText Text:="Baseline Name: "
Selection.Font.bold = wdToggle
Selection.TypeText Text:=baselineName
Selection.TypeParagraph
Selection.Font.bold = wdToggle
Selection.TypeText Text:="Baseline Description: "
Selection.Font.bold = wdToggle
Selection.TypeText Text:=baselineDescription
Selection.TypeParagraph
'RMsis graph api to fetch Requirements present in Baseline with id=5 i.e beta
rmsisApiString = "{getTargetRelationshipEntities(name:BASELINE_REQUIREMENT sourceId:5)}"
responseText = GetRequestfromRMsis(rmsisApiString)
Set JSON = ParseJson(responseText)
Dim requirementIds As Collection, requirementId As Integer
Set requirementIds = JSON("data")("getTargetRelationshipEntities")
Dim requirementKey, requirementSummary As String
Selection.Font.underline = wdUnderlineSingle
Selection.TypeText Text:="Requirements Present in Baseline:"
Selection.Font.underline = wdUnderlineNone
Selection.TypeParagraph
For i = 1 To requirementIds.Count
requirementId = requirementIds(i)
rmsisApiString = "{getRequirementById(id:reqId){key summary}}"
rmsisApiString = Replace(rmsisApiString, "reqId", requirementId)
responseText = GetRequestfromRMsis(rmsisApiString)
Set JSON = ParseJson(responseText)
requirementKey = JSON("data")("getRequirementById")("key")
requirementSummary = JSON("data")("getRequirementById")("summary")
Selection.Font.bold = wdToggle
Selection.TypeText Text:=vbTab & requirementKey
Selection.Font.bold = wdToggle
Selection.TypeText Text:=" " & requirementSummary
Selection.TypeParagraph
Next i
End Sub
'Function to fetch details using RMsis graph APIs
Function GetRequestfromRMsis(rmsisApiString) As String
Dim stringURL As String
Dim auth
auth = "(YWRtaW46YWRtaW4=)"
Set restRequest = CreateObject("Msxml2.ServerXMLHTTP.6.0")
stringURL = "http://10.0.1.10:8080/rest/service/latest/rmsis/graphql?query="
restRequest.Open "GET", stringURL & rmsisApiString, False
restRequest.setRequestHeader "Content-Type", "application/json"
restRequest.setRequestHeader "Authorization", "Basic " & auth
restRequest.send
If restRequest.Status = "401" Then
MsgBox "Not authorized"
Else
GetRequestfromRMsis = restRequest.responseText
End If
End Function